Using Visual Studio Code with PHP and Xdebug
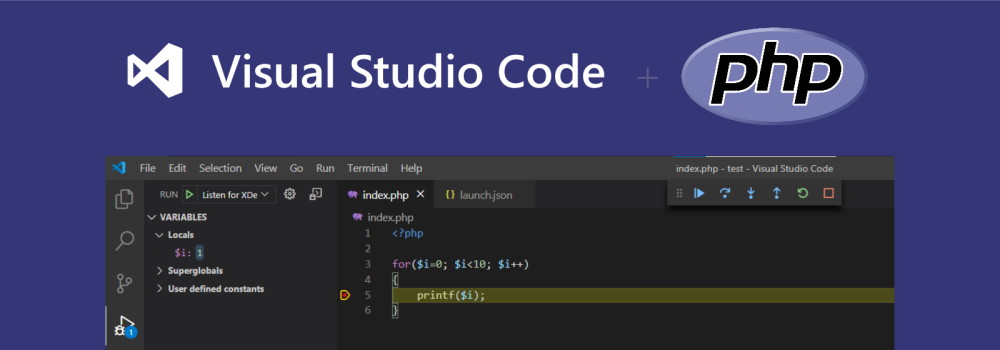
This post describes how to set up, run, and debug PHP code with Visual Studio Code running on Windows.
What do we need?
Compared to some other popular programming languages for the Web, PHP is missing some tools, so I created a shopping list to make the experience more complete.
Basically what we need is:
- the PHP runtime itself,
- a modern code editor with syntax highlighting,
- a debugger connected to our code editor,
- and a (development) webserver.
Runtime (PHP)
We start with the PHP runtime. The PHP runtime will pars en execute our .php
files and outputs the HTML (or anything you want) back to you.
- Download PHP version 7.4 (VC15 x64 Non Thread Safe) and extract the archive to
c:\php\
. - Add the folder
c:\php\
to your path, so we can easily access it.setx path "%path%;C:\php\"
Recent versions of PHP are built with Visual Studio 2019. You need to install the Visual C++ Redistributable for Visual Studio 2019 before PHP can run.
winget install "Microsoft Visual C++ 2015-2019 Redistributable (x64)"
- Now we can run PHP
php -v
and see if it works. It should show you the version number and copyright information.
Debugger (Xdebug)
To debug PHP code, we need to add an extension Xdebug to PHP.
- Download Xdebug 2.9 for PHP 7.4 (VC15 64 bit) and place the DLL into the folder
C:\php\ext
. - In the folder
c:\php\
rename the filephp.ini-development
tophp.ini
. - Add to the following lines to the file
php.ini
to tell PHP to use Xdebug:
|
|
- Check if Xdebug is part of PHP, use the
php -v
command again, and see if you get the response with Xdebug v2.9.6.
Webserver (IIS Express)
When creating web applications/sites, we also need a webserver. PHP does not have a development web server, so we need to use something else to serve our pages. Under Windows, it is common to use Microsoft Internet Information Services (IIS). We are using the lightweight (nonproduction) version IIS Express.
- Download IIS 10 Express.
- Start the setup and install it on your system
- Run IIS for the first time and quit (we need the initial configuration files)
|
|
- We need to tell IIS to use the PHP runtime (using fastCGI) and associate
.php
files with it. We also tell IIS to useindex.php
as a starting point:
|
|
Code editor (VSCode)
Visual Studio Code is a cross-platform, free, and opensource code editor. I use it for most of my projects.
-
Download Visual Studio Code or use WinGet
winget install "Visual Studio Code"
-
Run the setup and install it on your system
-
Add the two following extensions to Visual Studio Code:
-
In your Visual Studio Code project, go to the debugger and hit the little gear icon ⚙️ and choose PHP. A new launch configuration file is created for you
launch.json
. This will start listening on the specified port (by default 9000) for Xdebug. Every time you make a request with a browser to your web server, Xdebug will connect, and you can stop on breakpoints, exceptions, etc.
More information how to use the debugger in Visual Studio Code, you can read here https://code.visualstudio.com/Docs/editor/debugging
Visual Studio Code User Settings
To configure Visual Studio Code to use PHP, add the following line to the user configuration.
|
|
You now ready to develop, run, and debug PHP code on Windows.